Description
Supported Script Types: Interface Scripts • Client Entity Scripts • Avatar Scripts • Server Entity Scripts • Assignment Client Scripts
TheVec3
API provides facilities for generating and manipulating 3-dimensional vectors. Vircadia uses a
right-handed Cartesian coordinate system where the y-axis is the "up" and the negative z-axis is the "front" direction.
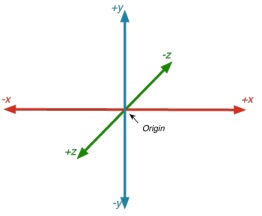
Properties
Name | Type | Summary |
---|---|---|
UNIT_X | Vec3 |
|
UNIT_Y | Vec3 |
|
UNIT_Z | Vec3 |
|
UNIT_NEG_X | Vec3 |
|
UNIT_NEG_Y | Vec3 |
|
UNIT_NEG_Z | Vec3 |
|
UNIT_XY | Vec3 |
|
UNIT_XZ | Vec3 |
|
UNIT_YZ | Vec3 |
|
UNIT_XYZ | Vec3 |
|
FLOAT_MAX | Vec3 |
|
FLOAT_MIN | Vec3 |
|
ZERO | Vec3 |
|
ONE | Vec3 |
|
TWO | Vec3 |
|
HALF | Vec3 |
|
RIGHT | Vec3 |
|
UP | Vec3 |
|
FRONT | Vec3 |
|
Methods
Name | Return Value | Summary |
---|---|---|
cross
|
Vec3 |
Calculates the cross product of two vectors. |
distance
|
number |
Calculates the distance between two points. |
dot
|
number |
Calculates the dot product of two vectors. |
equal
|
boolean |
Tests whether two vectors are equal. Note: The vectors must be exactly equal in order for |
fromPolar
|
Vec3 |
Calculates the coordinates of a point from polar coordinate transformation of the unit z-axis vector. |
fromPolar
|
Vec3 |
Calculates the unit vector corresponding to polar coordinates elevation and azimuth transformation of the unit z-axis vector. |
getAngle
|
number |
Calculates the angle between two vectors. |
length
|
number |
Calculates the length of a vector |
mix
|
Vec3 |
Calculates a linear interpolation between two vectors. |
multiply
|
Vec3 |
Multiplies a vector by a scale factor. |
multiply
|
Vec3 |
Multiplies a vector by a scale factor. |
multiplyQbyV
|
Vec3 |
Rotates a vector. |
multiplyVbyV
|
Vec3 |
Multiplies two vectors. |
normalize
|
Vec3 |
Normalizes a vector so that its length is |
orientedAngle
|
number |
Calculates the angle of rotation from one vector onto another, with the sign depending on a reference vector. |
print
|
None |
Prints the vector to the program log, as a text label followed by the vector value. |
reflect
|
Vec3 |
Calculates the reflection of a vector in a plane. |
subtract
|
Vec3 |
Calculates one vector subtracted from another. |
sum
|
Vec3 |
Calculates the sum of two vectors. |
toPolar
|
Vec3 |
Calculates polar coordinates (elevation, azimuth, radius) that transform the unit z-axis vector onto a point. |
withinEpsilon
|
boolean |
Tests whether two vectors are equal within a tolerance. Note: It is often better to use this function than equal. |
Method Details
(static) cross( v1, v2 ) → {Vec3}
Returns: The cross product of v1 and v2 .
|
|||||||||
---|---|---|---|---|---|---|---|---|---|
Calculates the cross product of two vectors. Parameters
ExampleThe cross product of x and y unit vectors is the z unit vector.
|
(static) distance( p1, p2 ) → {number}
Returns: The distance between the two points. |
|||||||||
---|---|---|---|---|---|---|---|---|---|
Calculates the distance between two points. Parameters
ExampleThe distance between two points is aways positive.
|
(static) dot( v1, v2 ) → {number}
Returns: The dot product of v1 and v2 .
|
|||||||||
---|---|---|---|---|---|---|---|---|---|
Calculates the dot product of two vectors. Parameters
ExampleThe dot product of vectors at right angles is
|
(static) equal( v1, v2 ) → {boolean}
Returns: true if the two vectors are exactly equal, otherwise false .
|
|||||||||
---|---|---|---|---|---|---|---|---|---|
Tests whether two vectors are equal. Note: The vectors must be exactly equal in order for Parameters
ExampleVectors are only equal if exactly the same.
|
(static) fromPolar( polar ) → {Vec3}
Returns: The coordinates of the point. |
||||||
---|---|---|---|---|---|---|
Calculates the coordinates of a point from polar coordinate transformation of the unit z-axis vector. Parameters
ExamplePolar coordinates to Cartesian.
|
(static) fromPolar( elevation, azimuth ) → {Vec3}
Returns: Unit vector for the direction specified by elevation and azimuth .
|
|||||||||
---|---|---|---|---|---|---|---|---|---|
Calculates the unit vector corresponding to polar coordinates elevation and azimuth transformation of the unit z-axis vector. Parameters
ExamplePolar coordinates to Cartesian coordinates.
|
(static) getAngle( v1, v2 ) → {number}
Returns: The angle between the two vectors, in radians. |
|||||||||
---|---|---|---|---|---|---|---|---|---|
Calculates the angle between two vectors. Parameters
ExampleCalculate the angle between two vectors.
|
(static) length( v ) → {number}
Returns: The length of the vector. |
||||||
---|---|---|---|---|---|---|
Calculates the length of a vector Parameters
|
(static) mix( v1, v2, factor ) → {Vec3}
Returns: The linear interpolation between the two vectors: (1 - factor) * v1 + factor * v2 .
|
||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|
Calculates a linear interpolation between two vectors. Parameters
ExampleLinear interpolation between two vectors.
|
(static) multiply( v, scale ) → {Vec3}
Returns: The vector with each ordinate value multiplied by the scale .
|
|||||||||
---|---|---|---|---|---|---|---|---|---|
Multiplies a vector by a scale factor. Parameters
|
(static) multiply( scale, v ) → {Vec3}
Returns: The vector with each ordinate value multiplied by the scale .
|
|||||||||
---|---|---|---|---|---|---|---|---|---|
Multiplies a vector by a scale factor. Parameters
|
(static) multiplyQbyV( q, v ) → {Vec3}
Returns: v rotated by q .
|
|||||||||
---|---|---|---|---|---|---|---|---|---|
Rotates a vector. Parameters
ExampleRotate the negative z-axis by 90 degrees about the x-axis.
|
(static) multiplyVbyV( v1, v2 ) → {Vec3}
Returns: A vector formed by multiplying the ordinates of two vectors: { x: v1.x * v2.x, y: v1.y * v2.y,
z: v1.z * v2.z } .
|
|||||||||
---|---|---|---|---|---|---|---|---|---|
Multiplies two vectors. Parameters
ExampleMultiply two vectors.
|
(static) normalize( v ) → {Vec3}
Returns: The vector normalized to have a length of 1 .
|
||||||
---|---|---|---|---|---|---|
Normalizes a vector so that its length is Parameters
ExampleNormalize a vector.
|
(static) orientedAngle( v1, v2, ref ) → {number}
Returns: The angle of rotation from the first vector to the second, in degrees. The value is positive if the rotation axis aligns with the reference vector (has a positive dot product), otherwise the value is negative. |
||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|
Calculates the angle of rotation from one vector onto another, with the sign depending on a reference vector. Parameters
ExampleCompare
|
(static) print( label, v ) | |||||||||
---|---|---|---|---|---|---|---|---|---|
Prints the vector to the program log, as a text label followed by the vector value. Parameters
ExampleTwo ways of printing a label and vector value.
|
(static) reflect( v, normal ) → {Vec3}
Returns: The vector reflected in the plane given by the normal. |
|||||||||
---|---|---|---|---|---|---|---|---|---|
Calculates the reflection of a vector in a plane. Parameters
ExampleReflect a vector in the x-z plane.
|
(static) subtract( v1, v2 ) → {Vec3}
Returns: The second vector subtracted from the first. |
|||||||||
---|---|---|---|---|---|---|---|---|---|
Calculates one vector subtracted from another. Parameters
|
(static) sum( v1, v2 ) → {Vec3}
Returns: The sum of the two vectors. |
|||||||||
---|---|---|---|---|---|---|---|---|---|
Calculates the sum of two vectors. Parameters
|
(static) toPolar( p ) → {Vec3}
Returns: Vector of polar coordinates for the point: x elevation rotation about the x-axis in
radians, y azimuth rotation about the y-axis in radians, and z radius.
|
||||||
---|---|---|---|---|---|---|
Calculates polar coordinates (elevation, azimuth, radius) that transform the unit z-axis vector onto a point. Parameters
ExamplePolar coordinates for a point.
|
(static) withinEpsilon( v1, v2, epsilon ) → {boolean}
Returns: true if the distance between the points represented by the vectors is less than or equal
to epsilon , otherwise false .
|
||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|
Tests whether two vectors are equal within a tolerance. Note: It is often better to use this function than equal. Parameters
ExampleTesting vectors for near equality.
|